Using Per-Customer Template Overrides
Template Overrides
When to override
Adjusting template column behavior on a per-customer basis (eg. picklist options).
If you are using our SDK to initialize your importer, pass in templateOverrides as part of the OneSchemaLaunchParams
.
If you are using the API, pass in template_overrides
to the create embedded session endpoint.
The format for templateOverrides
is OneSchemaTemplateOverrides
documented below. You can also find the TypeScript types here.
OneSchemaTemplateOverrides
Key | Type | Description |
---|---|---|
columns_to_add | OneSchemaTemplateColumnToAdd[] | Array of columns to add to the template. |
columns_to_update | OneSchemaTemplateColumnToUpdate[] | Array of columns to update in the template. OneSchema identifies columns via the key and replaces the properties of the column with those provided in the template overrides. |
columns_to_remove | OneSchemaTemplateColumnToRemove[] | Array of columns to remove from the template. |
validation_hooks_to_add | OneSchemaValidationHooksToAdd[] | Array of validation hook to add to the template. |
OneSchemaTemplateColumnToAdd
Key | Type | Required | Description |
---|---|---|---|
key | string | true | Unique key identifier for a given template column |
label | string | true | Display label of the template column for end users |
data_type | string | Data type for the template column (eg. "PICKLIST" or "NUMBER") | |
validation_options | Object | Additional validation properties on top of specific data types (full list below) | |
description | string | Description displayed to end user | |
is_custom | boolean | Whether the template column should act as a Custom Column | |
is_required | boolean | Cells in this column cannot have blank values. | |
is_unique | boolean | All values in the column must be unique | |
is_locked | boolean | Users will not be able to edit data mapped to this column on the Review & finalize step. | |
letter_case | string | Casing validation for the column (eg. "LETTER_CASE_UPPER", "LETTER_CASE_LOWER", or "LETTER_CASE_TITLE") | |
min_char_limit | integer | Min number of characters | |
max_char_limit | integer | Max number of characters | |
delimiter | string | Delimiter character to be used for separating multi-value fields. | |
must_exist | boolean | Column must be mapped | |
default_value | string | Automatically replace empty cells with provided default value | |
mapping_hints | string[] | Array of sheet column names to automatically map this column to |
OneSchemaTemplateColumnToUpdate
Key | Type | Required | Description |
---|---|---|---|
key | string | true | Unique key identifier for a given template column |
label | string | Display label of the template column for end users | |
data_type | string | Data type for the template column (eg. "PICKLIST" or "NUMBER") | |
validation_options | Object | Additional validation properties on top of specific data types (full list below) | |
description | string | Description displayed to end user | |
is_custom | boolean | Whether the template column should act as a Custom Column | |
is_required | boolean | Cells in this column cannot have blank values. | |
is_unique | boolean | All values in the column must be unique | |
is_locked | boolean | Users will not be able to edit data mapped to this column on the Review & finalize step. | |
letter_case | string | Casing validation for the column (eg. "LETTER_CASE_UPPER", "LETTER_CASE_LOWER", or "LETTER_CASE_TITLE") | |
min_char_limit | integer | Min number of characters | |
max_char_limit | integer | Max number of characters | |
delimiter | string | Delimiter character to be used for separating multi-value fields. | |
must_exist | boolean | Column must be mapped | |
default_value | string | Automatically replace empty cells with provided default value | |
mapping_hints | string[] | Array of sheet column names to automatically map this column to |
Validation Options by Data Type
Data Type | Validation Options | Description |
---|---|---|
ALPHABETICAL | allow_spaces?: boolean allow_special?: boolean | Optional. Controls whether spaces and special characters are allowed |
BOOLEAN | true_label: string false_label: string | Required. Labels for true and false values |
CUSTOM_REGEX | regex: string error_message: string | Required. Custom regex pattern and error message |
DATE_ISO DATE_MDY DATE_DMY DATE_YMD DATE_DMMMY DATETIME_ISO DATETIME_MDYHM DATETIME_DMYHM UNIX_TIMESTAMP | input_date_order?: "dmy" | "mdy" | "ymd" | Optional. Specifies the order of date components for ambiguous dates |
ENUM_COUNTRY | format: "name" | "code2" | "code3" | Optional. Format for country values. Defaults to "name" |
ENUM_US_STATE_TERRITORY | format: "name" | "code" variant_set_mods?: ["include_dc"] | ["include_territories"] | ["include_dc", "include_territories"] | Optional. Format for US state/territory values. Defaults to format:"name" |
FILE_NAME | extensions: string[] | Optional. List of allowed file extensions |
MONEY | currency_symbol: "dollar" | "euro" | "pound" | "yen" | Optional. Currency symbol to use. Defaults to "dollar" |
NUMBER | max_num?: number min_num?: number only_int?: boolean allow_thousand_separators?: boolean num_decimals?: number | Optional. Numeric constraints |
PICKLIST | picklist_options: Array<{ value: string, color?: string }> | Required. List of valid options with optional colors |
The following data types do not support validation options:
CURRENCY_CODE
DOMAIN
EAN
EMAIL
IANA_TIMEZONE
IMEI
JSON
LOCATION_POSTALCODE
PERCENTAGE
PHONE_NUMBER
SSN_MASKED
SSN_UNMASKED
TEXT
TIME_HHMM
UNIT_OF_MEASURE
UPC_A
URL
US_PHONE_NUMBER_EXT
UUID
OneSchemaTemplateColumnToRemove
Key | Type | Required | Description |
---|---|---|---|
key | string | true | Unique key identifier for a given template column |
OneSchemaValidationHooksToAdd
Key | Type | Required | Description |
---|---|---|---|
name | string | true | Name of the validation hook. |
url | string | true | The URL where the validation hook will be called. Environment variables can be used when setting the url. |
column_keys | string[] | Columns to be sent in the validation hook. | |
custom_column_keys | string[] | Custom columns to be sent in the validation hook | |
hook_type | string | One of row or column . See Validation Webhook for more details. Defaults to row if not provided. | |
secret_key | string | If a secret key is provided, the secret key will be used as the basic auth password. | |
batch_size | integer | Batch size for row hooks. Defaults to 1000 if not provided. |
Sample Usage
Below is an example use case for a number of overrides to a sample template with key crm_test
. The following actions are taken:
- Create a new column
account_executive
which is marked as required. - Replace the valid picklist values on column
segment
with["A", "B"]
- Update column
phone_number
to be both required and unique - Update the description and label of column
metadata
- Remove the column
lead_source
const importer = oneSchemaImporter({
clientId: "CLIENT_ID",
userJwt: "USER_JWT",
templateKey: "crm_test",
templateOverrides: {
columns_to_add: [
{
key: "account_executive",
label: "Account Executive",
data_type: "TEXT",
is_required: true
}
],
columns_to_update: [
{
key: "segment",
validation_options: {
values: ["A", "B"]
},
},
{
key: "phone_number",
is_required: true,
is_unique: true,
},
{
key: "metadata",
label: "Customer Info",
description: "Provide any additional metadata about the customer as freeform text."
}
],
columns_to_remove: [
{
key: "lead_source",
},
],
validation_hooks_to_add: [
{
name: "Phone number validator",
url: "{{base_url}}/oneschema-webhooks/phone-number-validator",
column_keys: ["phone_number"]
}
]
},
})
Picklist Overrides
Picklist overrides are a subset of templateOverrides
. For picklist columns, you must configure the options via validation_options
. If you only want to update the values themselves, you can pass in values
as an array of strings. OneSchema will automatically assign colors to your options in reverse rainbow order (from purple to red).
validation_options: {
values: ["Seedling","Still growing", "Fully grown"]
},
However, if you would like to specify the color, description, and/or alternative picklist names, you should instead pass in picklist_options
. This should be an array of OneSchemaPicklistOption
objects
OneSchemaPicklistOption
Key | Type | Description |
---|---|---|
value | string | Value of the picklist option |
color | string | Color of the option, either one of the OneSchemaPicklistColors or a 6-digit hex code |
description | string | Description of the picklist option to be displayed in option dropdowns. |
alternative_names | string[] | List of alternative names for the specific picklsit option. If uploaded values match any of the alternative names, the picklist option will be matched automatically. |
Picklist Color Overrides
Colors should either be one of the OneSchemaPicklistColors
or, you can simply pass in a hex code (eg. #FF00AA
).
OneSchemaPicklistColors
Token Name | Hex code |
---|---|
ColorSelectorPurple100 | # 722ED1 |
ColorSelectorBluePurple100 | # 2F7AEB |
ColorSelectorBlue100 | # 40A9FF |
ColorSelectorGreenBlue100 | # 33C18E |
ColorSelectorGreen100 | # 52C41A |
ColorSelectorYellow100 | # EFC600 |
ColorSelectorOrange100 | # EF8F00 |
ColorSelectorRed100 | # F5222D |
ColorSelectorPink100 | # EB2F96 |
ColorSelectorGray100 | # 595959 |
Picklist Descriptions
If you would like to provide additional information to your customers, you can specify string descriptions which will be displayed below each option.
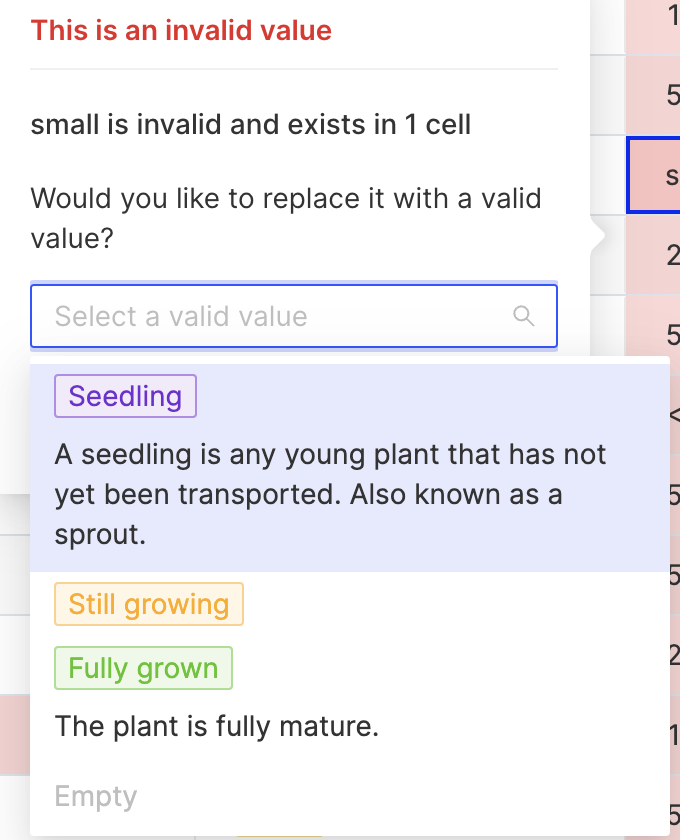
Sample Usage
const importer = oneSchemaImporter({
clientId: "CLIENT_ID",
userJwt: "USER_JWT",
templateKey: "products",
templateOverrides: {
columns_to_update: [
{
key: "size",
validation_options: {
picklist_options: [
{
value: "Seedling",
color: "ColorSelectorPurple100",
alternative_names: ["Sprout"],
description: "A seedling is any young plant that has not yet been transported. Also known as a sprout."
},
{
value:"Still growing",
color:"#FFAA00",
},
{
value:"Fully grown",
color:"ColorSelectorGreen100"
}
]
},
}
]
},
})
Updated 4 months ago